19. 删除链表的倒数第 N 个结点
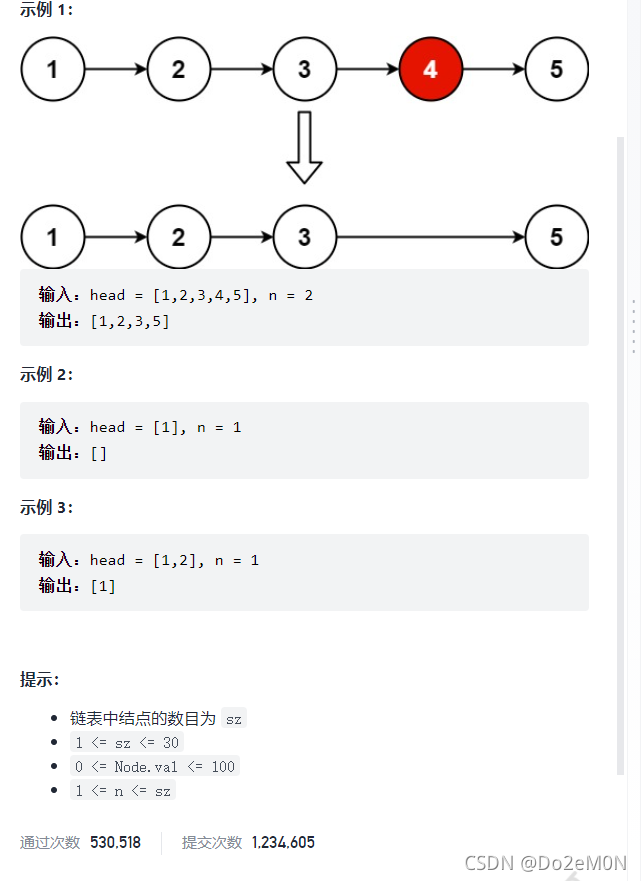
题目——[链接](19. 删除链表的倒数第 N 个结点 - 力扣(LeetCode) (leetcode-cn.com))
遍历统计方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| class Solution { public: ListNode* removeNthFromEnd(ListNode* head, int n) { if(!head) { return NULL; } int count = 0; ListNode* tempnode = head; ListNode* temp = NULL; int prev = 0; while(tempnode) { count++; tempnode = tempnode->next; }
tempnode = head; if(count == n) { temp = head; head = head->next; delete temp; return head; } while(prev != count -n) { prev++; if(prev == count-n) { break; } tempnode = tempnode->next; } temp =tempnode->next; tempnode->next = temp->next; delete temp;
return head;
} };
|
哨兵结点
在上面方法的基础上加入一个哨兵结点。
哨兵结点的next指向head。
(LeetCode中的head都是带数据的)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| class Solution { public: ListNode* removeNthFromEnd(ListNode* head, int n) { if(!head) { return NULL; } int count = 0; int prev = -1; ListNode* temp = NULL; ListNode* tempHead = new ListNode; ListNode* tempnode = head;
tempHead->next = head; while(tempnode) { count++; tempnode = tempnode->next; }
tempnode = tempHead; while(prev !=count-n) { prev++; if(prev == count-n) { break; } tempnode =tempnode->next; }
temp = tempnode->next; tempnode->next= temp->next; delete temp; return tempHead->next;
} };
|
快慢指针+哨兵结点
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| class Solution { public: ListNode* removeNthFromEnd(ListNode* head, int n) { if(!head) { return NULL; } ListNode* tempHead = new ListNode; ListNode* temp = NULL; int count = 0; ListNode* slow = tempHead; ListNode* fast =tempHead; tempHead ->next = head; while(count != n) { fast = fast->next; count++; } while(fast->next) { fast = fast->next; slow = slow->next; } temp = slow->next; slow->next = temp->next;
delete temp; return tempHead->next; } };
|