相关视频——黑马程序员C++(264-281)
基础部分(1-83)——如果你准备学习C++,并且有C语言的基础,我希望你能简单的过一遍知识点。
核心部分(84-146)——C++核心编程部分
案例1(147-166)——职工管理系统——C++实现职工管理系统
进阶部分(167-263)——C++提高编程部分
演讲比赛流程管理系统
演讲比赛程序需求
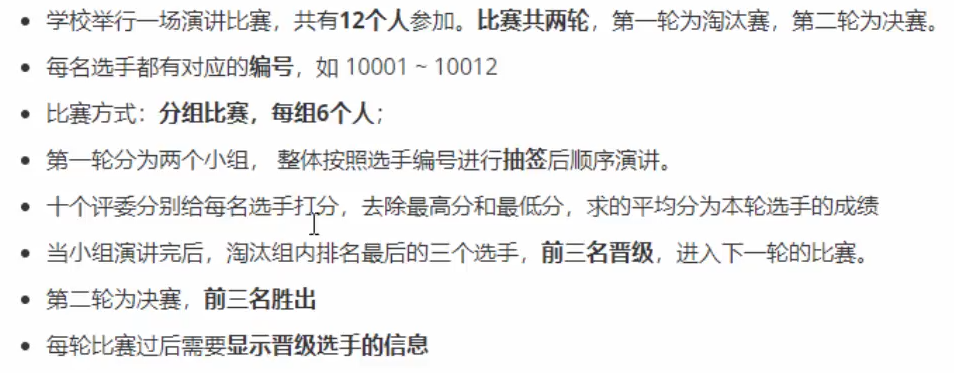
程序功能

代码实现
Speaker.h
1 2 3 4 5 6 7 8 9
| #pragma once #include<iostream> using namespace std; class Speaker { public: string m_Name; double m_Score[2]; };
|
SpeechManager.h
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| #pragma once #include<iostream> #include<vector> #include<map> #include"Speaker.h" #include<algorithm> #include<deque> #include<functional> #include<numeric> #include<string> #include<fstream> using namespace std;
class SpeechManager { public: SpeechManager();
void Show_Menu();
void ExitSystem();
void InitSpeech();
void CreatSpeaker();
void StartSpeech();
void SpeechDraw();
void SpeechContest();
void ShowScore();
void SaveRecord();
void LoadRecord();
void ShowRecord();
bool FileIsEmpyt;
map<int, vector<string>>m_Record; ~SpeechManager(); void ClearRecord();
vector<int>v1; vector<int>v2; vector<int>vVictory; map<int, Speaker>m_Speaker;
int m_Index;
};
|
SpeechManager.cpp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362
| #include"SpeechManager.h"
SpeechManager::SpeechManager() { this->InitSpeech(); this->CreatSpeaker(); this->LoadRecord();
}
void SpeechManager::Show_Menu() { cout << "*********************" << endl; cout << "****欢迎参加演讲比赛" << endl; cout << "*****1.开始比赛*****" << endl; cout << "*****2.查看记录*****" << endl; cout << "*****3.清空记录*****" << endl; cout << "*****0.退出程序*****" << endl; cout << "*********************" << endl; }
void SpeechManager::ExitSystem() { cout << "欢迎下次使用" << endl; exit(0); }
void SpeechManager::InitSpeech() { this->v1.clear(); this->v2.clear(); this->vVictory.clear(); this->m_Speaker.clear(); this->m_Index = 1;
this->m_Record.clear();
}
void SpeechManager::CreatSpeaker() { string NameSeed = "ABCDEFGHIJKL"; for (unsigned int i = 0; i < NameSeed.size(); i++) { string name = "选手"; name += NameSeed[i]; Speaker sp; sp.m_Name = name; for (int j = 0; j < 2; j++) { sp.m_Score[j] = 0; } this->v1.push_back(i + 10001);
this->m_Speaker.insert(make_pair(i + 10001, sp)); } }
void SpeechManager::SpeechDraw() { cout << "第" << this->m_Index << "轮比赛选手正在抽签" << endl; cout << "——————" << endl; if (this->m_Index == 1) { random_shuffle(v1.begin(), v1.end()); for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++) { cout << *it << " "; } cout << endl; } else { random_shuffle(v2.begin(), v2.end()); for (vector<int>::iterator it = v2.begin(); it != v2.end(); it++) { cout << *it << " "; } cout << endl; } }
void SpeechManager::StartSpeech() {
this->SpeechDraw(); this->SpeechContest(); this->ShowScore(); this->m_Index++; this->SpeechDraw(); this->SpeechContest(); this->ShowScore(); this->SaveRecord();
this->InitSpeech(); this->CreatSpeaker(); this->LoadRecord(); cout << "本届比赛已经结束!" << endl;
system("pause"); system("cls");
}
void SpeechManager::SpeechContest() { cout << "第" << this->m_Index << "轮比赛正式开始" << endl; multimap<double, int, greater<double>>groupScore;
int num = 0;
vector<int>v_Src; if (this->m_Index == 1) { v_Src = v1; } else { v_Src = v2; }
for (vector<int>::iterator it = v_Src.begin(); it != v_Src.end(); it++) { num++; deque<double>d; for (int i = 0; i < 10; i++) { double score = (rand() % 401 + 600) / 10.f; d.push_back(score); } sort(d.begin(), d.end(), greater<double>()); d.pop_front(); d.pop_back();
double sum = accumulate(d.begin(), d.end(), 0.0f); double avg = sum / (double)d.size();
this->m_Speaker[*it].m_Score[this->m_Index - 1] = avg;
groupScore.insert(make_pair(avg, *it)); if (num % 6 == 0) { cout << "第" << num /6<< "小组比赛名次" << endl; for (multimap<double, int, greater<double>>::iterator it = groupScore.begin(); it != groupScore.end(); it++) { cout << "编号: " << it->second << "姓名: " << this->m_Speaker[it->second].m_Name << "成绩: " << this->m_Speaker[it->second].m_Score[this->m_Index-1] << endl; } int count = 0; for (multimap<double, int, greater<double>>::iterator it = groupScore.begin(); it != groupScore.end() && count < 3; it++, count++) { if (this->m_Index == 1) { v2.push_back((*it).second); } else { vVictory.push_back((*it).second); } } groupScore.clear(); } } cout << "第" << this->m_Index << "轮比赛完毕" << endl; system("pause"); }
void SpeechManager::ShowScore() { cout << "第" << this->m_Index << "轮晋级选手如下" << endl; vector<int>v; if (this->m_Index == 1) { v = v2; } else { v = vVictory; }
for (vector<int>::iterator it = v.begin(); it != v.end();it++) { cout << "选手编号: " << *it << "姓名: " << this->m_Speaker[*it].m_Name << "得分: " << this->m_Speaker[*it].m_Score[this->m_Index - 1] << endl; } system("pause"); system("cls"); this->Show_Menu(); }
void SpeechManager::SaveRecord() { ofstream ofs; ofs.open("speech.csv", ios::out | ios::app); for (vector<int>::iterator it = vVictory.begin(); it != vVictory.end(); it++) { ofs << *it << "," << this->m_Speaker[*it].m_Score[1] << ","; } ofs << endl;
ofs.close(); cout << "记录已经完成" << endl;
this->FileIsEmpyt = false; }
void SpeechManager::LoadRecord() { ifstream ifs("speech.csv", ios::in); if (!ifs.is_open()) { this->FileIsEmpyt = true; ifs.close(); return; }
char ch; ifs >> ch; if (ifs.eof()) { cout << "文件为空" << endl; this->FileIsEmpyt = true; ifs.close(); return; }
this->FileIsEmpyt = false; ifs.putback(ch); string data; int index = 0; while (ifs >> data) { vector<string>v; int pos = -1; int start = 0; while (1) { pos = data.find(",", start); if (pos == -1) { break; } string temp = data.substr(start, pos - start); v.push_back(temp); start = pos + 1; } this->m_Record.insert(make_pair(index, v)); index++; } ifs.close();
}
void SpeechManager::ShowRecord() { if (this->FileIsEmpyt) { cout << "文件为或者文件不存在" << endl; } for (int i = 0; i < this->m_Record.size(); i++) { cout << "第" << i + 1 << "届 " << "冠军编号: " << this->m_Record[i][0] << "得分: " << this->m_Record[i][1] << " " << "亚军编号: " << this->m_Record[i][2] << "得分: " << this->m_Record[i][3] << " " << "季军编号: " << this->m_Record[i][4] << "得分: " << this->m_Record[i][5] << endl; }
system("pause"); system("cls"); }
void SpeechManager::ClearRecord() { cout << "是否确定清空文件?" << endl; cout << "1.是、2.否" << endl;
int select = 0; cin >> select; if (select == 1) { ofstream ofs("speech.csv",ios::trunc); ofs.close();
this->InitSpeech(); this->CreatSpeaker(); this->LoadRecord();
cout << "清空成功!" << endl; } system("pause"); system("cls"); }
SpeechManager::~SpeechManager() {
}
|
演讲比赛流程管理系统.cpp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| #include<iostream> #include<string> #include<ctime> #include"SpeechManager.h" using namespace std; int main(void) {
srand((unsigned int)time(NULL));
SpeechManager sm;
int choice = 0; while (1) { sm.Show_Menu(); cin >> choice; switch (choice) { case 1: sm.StartSpeech(); break; case 2: sm.ShowRecord(); break; case 3: sm.ClearRecord(); break; case 0: sm.ExitSystem(); break;
default: system("cls"); break; } }
system("pause"); return 0; }
|